Introduction
In the ever-evolving world of web and software development, staying ahead with modern practices is crucial for scalability, performance, and maintainability. One such approach that has gained widespread popularity in recent years is Component Driven Design (CDD). It represents a paradigm shift from monolithic development methods, focusing on the modularization of the codebase. By breaking down applications into smaller, reusable components, developers can create more manageable systems, increasing their efficiency and code maintainability.
This article explores the concept of component-driven design and development, providing a detailed analysis of its benefits, the best practices for adopting this methodology, and the tools and frameworks available. We will also delve into how CDD influences modern-day development processes and helps teams deliver better software. Whether you are a seasoned developer or a team manager, this technical guide will provide valuable insights into the future of web and software design.
Table of Contents
- Introduction
- Understanding Component Driven Design in Software Development
- The Core Principles of Component Driven Design
- Reusability and Modularity
- Encapsulation and Separation of Concerns
- Why Component Driven Design Matters in Modern Development
- Scalability in Development
- Maintainability and Reusability
- Best Practices for Component Driven Design
- Atomic Design and its Influence on Component Systems
- Building Small, Focused Components
- Testing Component Isolations
- Component Libraries and Design Systems
- Popular Component Libraries
- React and the Virtual DOM
- Vue.js for Simplicity and Flexibility
- Angular’s Complete Development Ecosystem
- Pros and Cons of Component Driven Development
- Benefits of Component Driven Development
- Challenges in Adopting Component Architecture
- Overcoming the Challenges
- Conclusion
Understanding Component Driven Design in Software Development
Component-driven design is the practice of breaking down the user interface (UI) or software into self-contained, reusable components. These components act as the building blocks of an application and can be independently developed, tested, and deployed. Component Driven Design (CDD) is a method where software and web applications are architected using small, modular components. Each component represents a distinct piece of functionality or UI element that can be independently developed, tested, and maintained. In essence, it promotes reusability and separation of concerns, where the User Interface (UI) and business logic are encapsulated in modular blocks.
This modular approach contrasts the traditional monolithic development style, where the entire application is built as a single unit. By dividing the application into smaller components, developers can maintain and update individual sections of the application without affecting the entire system. The rise of React, Vue.js, and Angular has solidified component-driven architecture in mainstream web development.
The Core Principles of Component-Driven Design

Component-driven design follows a structured and modular approach that builds applications by assembling individual components, each responsible for a specific UI or business logic. One of the core principles of component-driven design is modularity. Modularity means that each component is self-contained, with its data handling, UI logic, and business functionality encapsulated within it. This approach allows developers to easily swap or update components without causing side effects in other parts of the application.
Reusability and Modularity
Components in CDD are reusable across multiple projects or parts of the same project. They promote modularity by separating the application’s structure into isolated parts, which leads to cleaner, more maintainable code.
Decoupling Functionalities for Flexibility
By decoupling the codebase into distinct components, developers can easily modify or upgrade parts of the application without affecting other components. This makes the development process more flexible and scalable, especially in large-scale applications. Each component should be designed to serve a single, distinct purpose. This encapsulation ensures that each element is responsible for a particular task and can function independently. For example, a button component that handles user interactions and triggers specific actions, or a form component that manages data submission. By maintaining independent functionality, development teams can streamline the debugging, updating, and refactoring process, reducing the likelihood of introducing errors.
Example: Button Component
In most CDD practices, a button is not just a visual element but an entire self-contained piece of code that handles click events, styling, accessibility features, and more. This component could be reused across multiple parts of an application, and any updates made to it are reflected wherever the component is used, thus making the codebase more consistent and maintainable.
function Button({ label, onClick }) {
return <button onClick={onClick}>{label}</button>;
}
This simple React button component could be reused across multiple UI elements in a single application without writing redundant code.
Isolation of Business Logic
By isolating business logic within components, the system’s architecture becomes more maintainable. This encapsulation reduces the complexity of the codebase and promotes better development practices.
Encapsulation and Separation of Concerns
Encapsulation is one of the key tenets of component-driven design. Each component has its data, logic, and UI behavior, ensuring that concerns are separated.
Data Flow and State Management
The unidirectional flow of data in components improves the predictability of the application, making debugging and error tracing more manageable. Technologies like Redux, MobX, and Vuex are commonly used for efficient state management in component-based architectures.
Optimizing Performance via Lazy Loading
Components can be loaded lazily (i.e., only when needed), enhancing performance and reducing the initial load time. This is particularly useful in large-scale applications where performance can be affected by numerous UI components.
Why Component Driven Design Matters in Modern Development
In today’s fast-paced development environment, speed and efficiency are key metrics of success. The days of long development cycles and hard-to-maintain monolithic codebases are fading. Modern applications need to scale, evolve, and adapt quickly, and CDD provides an excellent solution to these challenges.
Scalability in Development
Component-driven design ensures that large-scale applications remain scalable by allowing teams to develop parts of the system independently. This methodology works particularly well with agile development, where features and components can be developed in parallel by different teams. As applications grow more complex, the modular structure of CDD allows for seamless scaling, with individual components being added or removed as needed without affecting the entire system.
Team Collaboration
Another advantage of CDD is the way it promotes team collaboration. By creating independent components, multiple developers or teams can work on different parts of the application simultaneously without conflicts. This also leads to better project organization, where each team is responsible for specific components or modules within the larger application.
Component Libraries for Better Collaboration
Incorporating component libraries like Material-UI for React or Vuetify for Vue.js enables teams to work from a prebuilt library of components, ensuring consistency and saving development time. These libraries provide ready-made components that can be customized to fit the branding and functionality needs of any project.
Benefits of Using Prebuilt Components
- Efficiency: Faster development with reusable components.
- Consistency: A standardized look and feel across the application.
- Accessibility: Built-in accessibility features that follow web standards.
Maintainability and Reusability
Component-driven design promotes maintainability by reducing code duplication. Once a component is built, it can be reused across multiple areas of the application, cutting down on redundant development and making maintenance easier. For example, a date picker component that is used in various forms across an application can be created once and referenced multiple times. Any updates to this component will be applied everywhere it is used.
Avoiding Code Duplication
In traditional development methodologies, teams often find themselves writing similar pieces of code repeatedly across different parts of an application. By using reusable components, teams can reduce code duplication, leading to cleaner, more efficient codebases that are easier to maintain.
Dynamic Components and Prop Passing
In frameworks like React or Vue, components can be dynamic, with data passed to them as props. This allows a single component to serve multiple purposes depending on the data it receives. For instance, a form component can be used for both user sign-up and product checkout by simply altering the data passed through props.
Code Example
function Form({ formType, submitHandler }) {
return (
<form onSubmit={submitHandler}>
<h1>{formType} Form</h1>
<input type="text" placeholder="Enter your details" />
<button type="submit">Submit</button>
</form>
);
}
This form component dynamically updates based on the formType prop, reducing the need for separate form components for each use case.
Best Practices for Component Driven Design
To get the most out of component-driven design, it’s important to follow industry best practices. These practices ensure that your components remain flexible, reusable, and easy to maintain. Below, we explore some common guidelines to keep in mind.
Atomic Design and its Influence on Component Systems
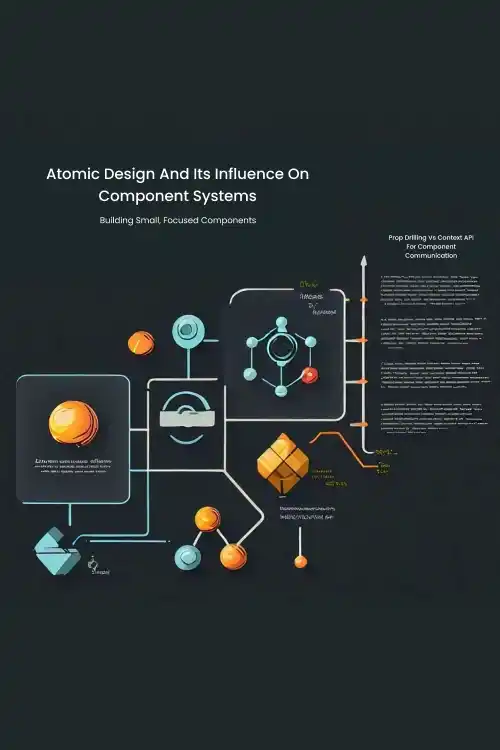
Atomic Design is a methodology introduced by Brad Frost that breaks down UI components into five hierarchical categories: atoms, molecules, organisms, templates, and pages. This design system works seamlessly with CDD by promoting smaller components that combine to form larger, more complex UI structures. It divides UI components into five distinct levels:
Level | Description |
---|---|
Atoms | The smallest, indivisible components (e.g., buttons, inputs). |
Molecules | Combinations of atoms that form a functional unit (e.g., form fields). |
Organisms | Complex UI elements, such as headers or cards, made up of multiple molecules. |
Templates | Layouts that define the structure of pages but without specific content. |
Pages | Fully rendered UI with content, built using templates. |
Building Small, Focused Components
Breaking components down to the smallest reusable parts, such as atoms, ensures maximum flexibility and reusability. These can be combined later to form more complex UI elements.
Prop Drilling vs Context API for Component Communication
In frameworks like React, data is passed to components via props. When you need to pass data deeply through the component tree, prop drilling becomes cumbersome. Using React Context API or libraries like Redux can simplify state management across multiple components.
Benefits of Atomic Design
- Consistency: Smaller, reusable components ensure uniformity.
- Scalability: Teams can easily scale their UI by building new molecules or organisms using existing atoms.
- Maintainability: Updating an atom will propagate changes across all UI elements using that atom.
Testing Component Isolations
Testing is a crucial part of CDD. Unit tests should be focused on isolated components to ensure that each unit behaves as expected. Frameworks like Jest, Mocha, and Enzyme offer powerful tools for component testing.
Snapshot Testing for Component Rendering
Snapshot testing allows you to capture the rendered output of components at a specific point in time. Any changes made to the components will trigger updates in the snapshot, making it easier to catch unintended side effects.
Integration Testing with Component Interaction
Integration tests simulate user interactions with components, ensuring they work together as intended. Tools like Cypress or Testing Library allow for end-to-end testing, improving the robustness of the application.
Component Libraries and Design Systems
Design systems and component libraries offer ready-made components for fast and consistent development. These can be adapted to your project’s needs and reduce the amount of code duplication.
Popular Component Libraries
React and the Virtual DOM
React’s Virtual DOM optimizes component rendering, improving performance by minimizing unnecessary updates to the actual DOM. This makes React an excellent choice for building highly dynamic web applications using CDD principles.
Vue.js for Simplicity and Flexibility
Vue.js offers a simpler learning curve compared to React, but still provides a powerful component-based architecture. Vue’s Single File Components (SFCs) package templates, styles, and logic into one file, making the development process straightforward.
Angular’s Complete Development Ecosystem
Angular provides a more opinionated, full-featured development framework with built-in dependency injection, services, and routing. Its component-based architecture and comprehensive tooling make it a strong choice for enterprise-level applications.
Some popular component libraries include:
Library | Framework | Features |
---|---|---|
Material-UI | React | Prebuilt Material Design components |
Vuetify | Vue | Material Design framework for Vue |
Ant Design | React/Angular | Enterprise-grade design system |
Bootstrap | General | CSS framework with UI components |
Building Custom Design Systems
For organizations that require a unique and consistent look and feel across multiple products, building a custom design system ensures that all components adhere to specific branding guidelines, reducing design debt over time.
Using Design Tokens for Consistency
Design tokens encapsulate design decisions like color, spacing, and typography in a way that can be reused across components. They ensure that your application’s components remain visually consistent throughout.
Pros and Cons of Component Driven Development
While component-driven design provides immense benefits, it also comes with its challenges. Understanding the trade-offs will help you make informed decisions when adopting this methodology.
Benefits of Component-Driven Development
- Reusability: Components can be reused across multiple projects or application parts, reducing duplication and saving development time.
- Consistency: With encapsulated logic and styling, component-driven applications ensure a consistent user experience.
- Scalability: Components can be developed independently, allowing teams to scale large applications effectively.
- Improved Testing: Testing is easier with components because you can isolate functionality and test it independently.
Challenges in Adopting Component Architecture
- Steep Learning Curve: New developers may struggle to understand the complexities of state management and component hierarchies.
- Performance Overhead: Overloading the application with too many components can introduce performance bottlenecks if not optimized.
- Code Duplication in Small Projects: In smaller applications, breaking things down into numerous components can feel unnecessary and lead to code duplication.
Overcoming the Challenges
Proper documentation, consistent development practices, and the use of performance optimizations like lazy loading can help overcome the challenges of component-driven development.
Conclusion
Component-driven design and development in web and software development offer a future-proof methodology to build scalable, maintainable, and reusable applications. From frameworks like React and Vue to design systems like Atomic Design, this methodology has become a cornerstone of modern development. Understanding the best practices, pros and cons, and the tools available will enable developers to deliver high-quality software at scale.
Discover Your Ideas With Us
Transform your business with our Web Development solutions. Achieve growth, innovation, and success. Collaborate with our skilled development team today to revolutionize your digital presence!